Creating Effective Livewire Components: A Step-by-Step Guide
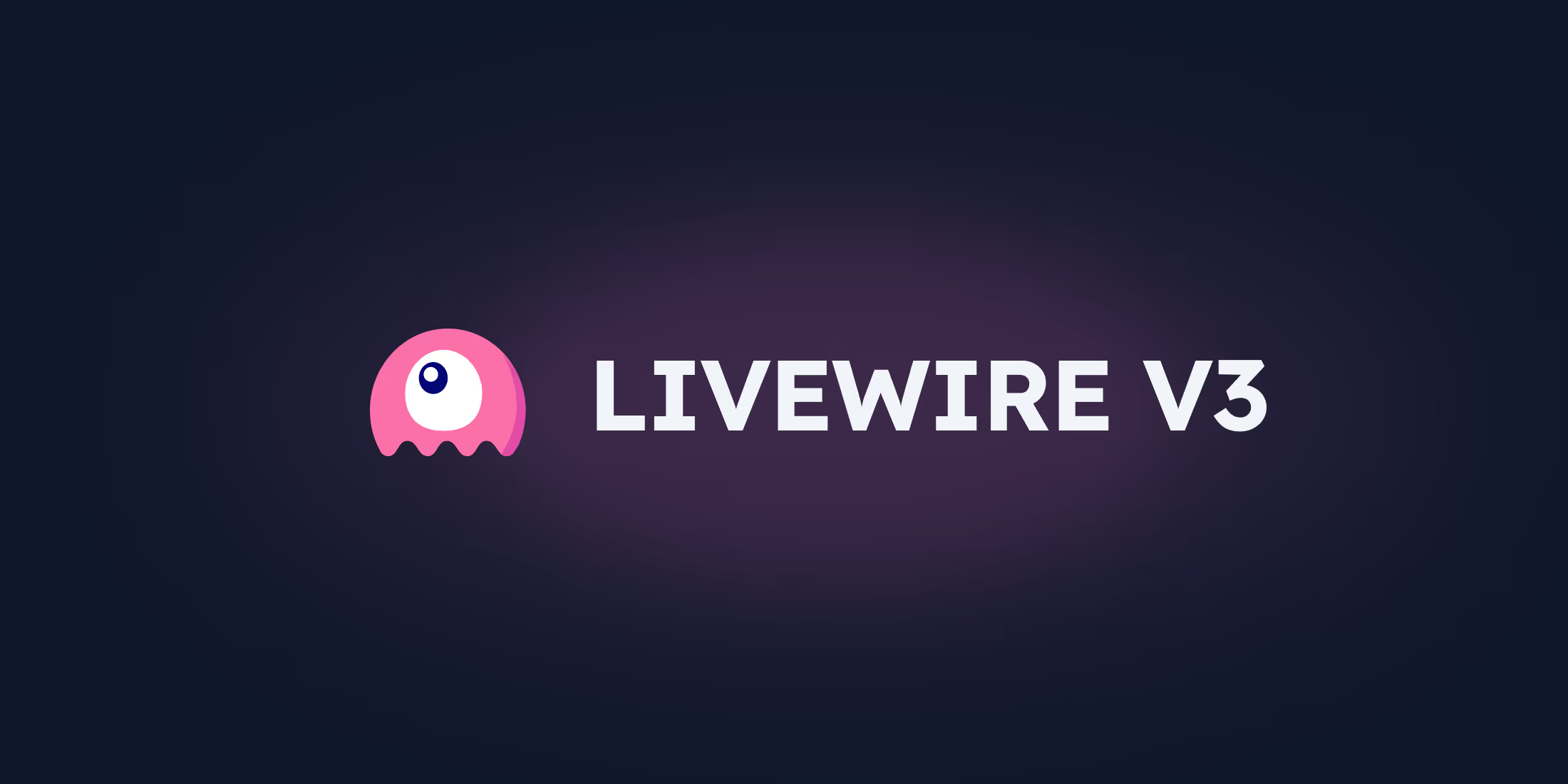
- 1. Plan Your Component Structure
- 2. Create the Component
- 3. Define Component Properties
- 4. Implement Core Methods
- 5. Create the View
- 6. Add Event Handling
- 7. Implement Real-time Updates
- 8. Testing Your Component
- Best Practices
- Common Pitfalls to Avoid
- Conclusion
Creating Effective Livewire Components: A Step-by-Step Guide
Building Livewire components in Laravel can streamline your development process and create dynamic interfaces without writing complex JavaScript. Here's a comprehensive guide on how to create effective Livewire components.
1. Plan Your Component Structure
Before writing any code, ask yourself these questions:
- What is the single responsibility of this component?
- What data does it need to manage?
- What actions will users perform?
- How will it interact with other components?
- What loading states need to be handled?
2. Create the Component
Use the Artisan command to generate your component:
php artisan make:livewire TaskList
This creates two files:
-
app/Http/Livewire/TaskList.php
-
resources/views/livewire/task-list.blade.php
3. Define Component Properties
Start with your component's properties:
class TaskList extends Component
{
// Public properties for two-way binding
public string $newTaskTitle = '';
public string $filter = 'all';
public array $tasks = [];
// Properties that need to be reset after actions
protected array $resetProperties = ['newTaskTitle'];
// Define validation rules
protected array $rules = [
'newTaskTitle' => 'required|min:3|max:255'
];
// URL query parameters
protected $queryString = [
'filter' => ['except' => 'all']
];
}
4. Implement Core Methods
Break down functionality into focused methods:
class TaskList extends Component
{
// ... properties from above ...
public function addTask(): void
{
$this->validate();
$this->tasks[] = [
'id' => Str::uuid(),
'title' => $this->newTaskTitle,
'completed' => false,
'created_at' => now()
];
$this->reset('newTaskTitle');
$this->emit('taskAdded');
}
public function toggleTask(string $taskId): void
{
$index = collect($this->tasks)->search(fn($task) => $task['id'] === $taskId);
if ($index !== false) {
$this->tasks[$index]['completed'] = !$this->tasks[$index]['completed'];
}
}
public function deleteTask(string $taskId): void
{
$this->tasks = collect($this->tasks)
->reject(fn($task) => $task['id'] === $taskId)
->values()
->all();
}
public function getFilteredTasksProperty(): array
{
return collect($this->tasks)
->when($this->filter === 'active', fn($tasks) => $tasks->where('completed', false))
->when($this->filter === 'completed', fn($tasks) => $tasks->where('completed', true))
->values()
->all();
}
}
5. Create the View
Design your component with user experience in mind:
<div>
{{-- Task Input Form --}}
<form wire:submit.prevent="addTask" class="mb-6">
<div class="flex gap-4">
<input
type="text"
wire:model.defer="newTaskTitle"
class="flex-1 rounded-lg border-gray-300 shadow-sm"
placeholder="What needs to be done?"
>
<button type="submit" class="px-4 py-2 bg-primary-600 text-white rounded-lg hover:bg-primary-700 transition-colors">
<span wire:loading.remove wire:target="addTask">Add Task</span>
<span wire:loading wire:target="addTask">Adding...</span>
</button>
</div>
@error('newTaskTitle')
<p class="mt-1 text-red-500 text-sm">{{ $message }}</p>
@enderror
</form>
{{-- Task Filters --}}
<div class="flex gap-4 mb-6">
@foreach(['all', 'active', 'completed'] as $filterOption)
<button
wire:click="$set('filter', '{{ $filterOption }}')"
class="px-3 py-1 rounded-full {{ $filter === $filterOption ? 'bg-primary-600 text-white' : 'bg-gray-100' }}"
>
{{ ucfirst($filterOption) }}
</button>
@endforeach
</div>
{{-- Tasks List --}}
<div class="space-y-2">
@forelse($this->filteredTasks as $task)
<div class="flex items-center justify-between p-4 bg-white rounded-lg shadow-sm">
<div class="flex items-center gap-3">
<input
type="checkbox"
wire:click="toggleTask('{{ $task['id'] }}')"
@checked($task['completed'])
class="rounded border-gray-300 text-primary-600 focus:ring-primary-500"
>
<span class="{{ $task['completed'] ? 'line-through text-gray-400' : '' }}">
{{ $task['title'] }}
</span>
</div>
<button
wire:click="deleteTask('{{ $task['id'] }}')"
class="text-red-500 hover:text-red-700"
>
<svg class="w-5 h-5" fill="none" stroke="currentColor" viewBox="0 0 24 24">
<path stroke-linecap="round" stroke-linejoin="round" stroke-width="2" d="M19 7l-.867 12.142A2 2 0 0116.138 21H7.862a2 2 0 01-1.995-1.858L5 7m5 4v6m4-6v6m1-10V4a1 1 0 00-1-1h-4a1 1 0 00-1 1v3M4 7h16" />
</svg>
</button>
</div>
@empty
<div class="text-center py-12 text-gray-500">
No tasks yet. Add one above!
</div>
@endforelse
</div>
</div>
6. Add Event Handling
Implement event listeners for component communication:
class TaskList extends Component
{
protected $listeners = [
'taskAdded' => 'handleTaskAdded',
'echo:tasks,TaskCreated' => 'handleBroadcastedTask'
];
public function handleTaskAdded(): void
{
$this->dispatch('notify', [
'message' => 'Task added successfully!',
'type' => 'success'
]);
}
public function handleBroadcastedTask($event): void
{
$this->tasks[] = $event['task'];
}
}
7. Implement Real-time Updates
Add real-time capabilities with Laravel Echo:
class TaskList extends Component
{
public function addTask(): void
{
$this->validate();
$task = [
'id' => Str::uuid(),
'title' => $this->newTaskTitle,
'completed' => false,
'created_at' => now()
];
$this->tasks[] = $task;
// Broadcast to other users
broadcast(new TaskCreated($task))->toOthers();
$this->reset('newTaskTitle');
$this->emit('taskAdded');
}
}
8. Testing Your Component
Write comprehensive tests:
class TaskListTest extends TestCase
{
/** @test */
public function can_add_task()
{
Livewire::test(TaskList::class)
->set('newTaskTitle', 'Test Task')
->call('addTask')
->assertHasNoErrors()
->assertSee('Test Task')
->assertEmitted('taskAdded');
}
/** @test */
public function cannot_add_task_without_title()
{
Livewire::test(TaskList::class)
->set('newTaskTitle', '')
->call('addTask')
->assertHasErrors(['newTaskTitle' => 'required']);
}
/** @test */
public function can_toggle_task_completion()
{
Livewire::test(TaskList::class)
->set('tasks', [[
'id' => 'test-id',
'title' => 'Test Task',
'completed' => false
]])
->call('toggleTask', 'test-id')
->assertSet('tasks.0.completed', true);
}
}
Best Practices
-
Keep Components Focused
- Single responsibility principle
- Break large components into smaller ones
- Use events for communication
-
Optimize Performance
- Use
wire:model.defer
for forms - Implement debounce for real-time search
- Avoid unnecessary re-renders
- Use
-
Handle Loading States
<button wire:loading.attr="disabled"> <span wire:loading.remove>Save</span> <span wire:loading>Saving...</span> </button>
-
Implement Error Handling
try { // Perform action } catch (\Exception $e) { session()->flash('error', 'Something went wrong!'); }
-
Use Computed Properties
public function getCompletedTasksCountProperty(): int { return collect($this->tasks)->where('completed', true)->count(); }
Common Pitfalls to Avoid
- Overloading Components: Keep them focused and manageable
- Skipping Loading States: Always handle loading for better UX
- Ignoring Error Handling: Implement proper error handling
- Missing Tests: Write comprehensive tests
- Poor Performance: Optimize renders and queries
Conclusion
Building effective Livewire components requires:
- Careful planning
- Clean code organization
- Proper error handling
- Comprehensive testing
- Performance optimization
- User experience consideration
Remember to:
- Keep components focused
- Handle edge cases
- Test thoroughly
- Consider user experience
- Document your code
Happy coding! ⚡️