Docker for Local Development: Best Practices and Tips
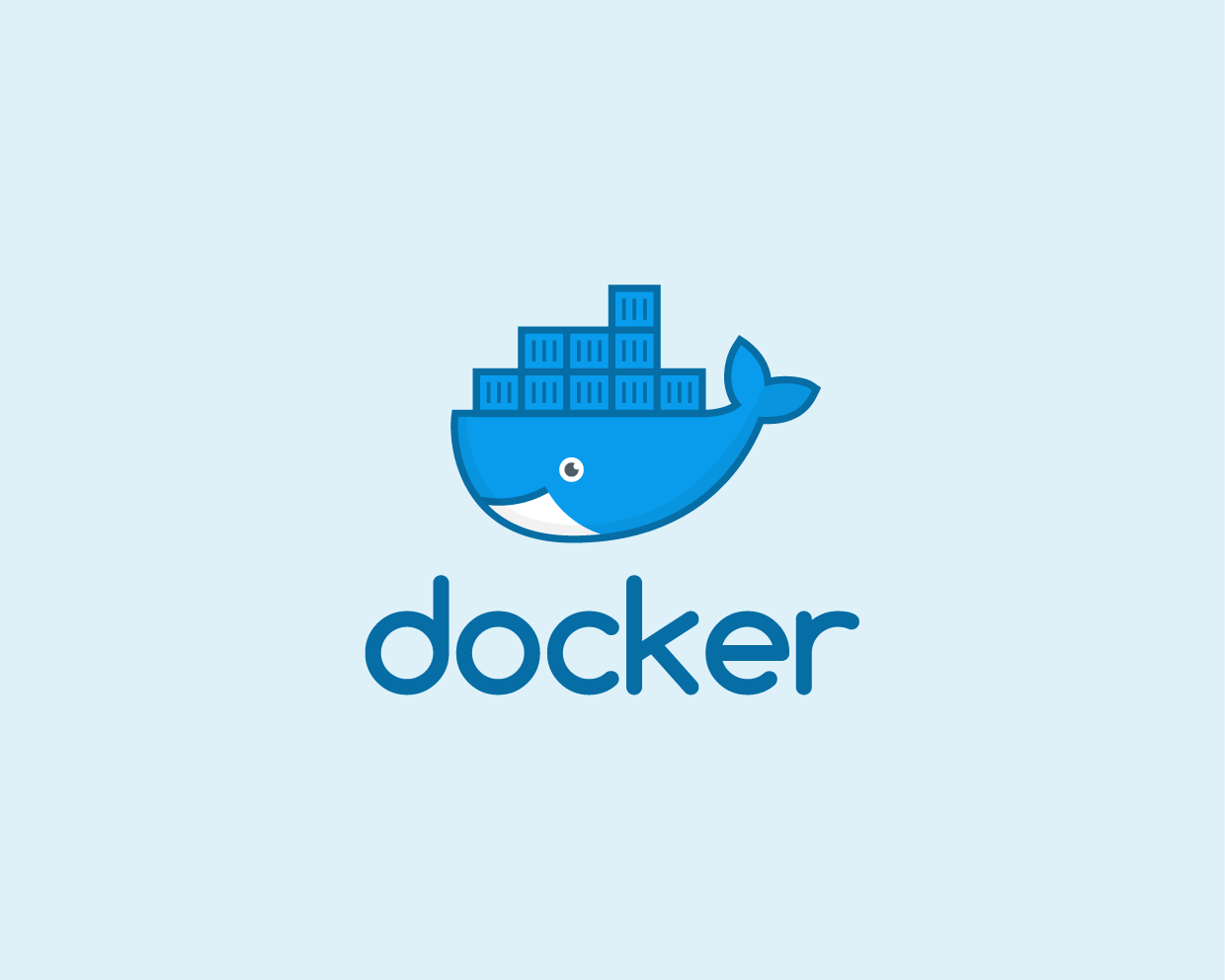
- 1. Docker Compose
- 2. Docker Volumes
- 3. Docker Networks
- 4. Docker Images
- 5. Docker Containers
- 6. Docker Registries
- 7. Docker Swarm
- 8. Docker Compose
- Best Practices
- Conclusion
Docker for Local Development: Best Practices and Tips
Docker is a powerful tool for local development that can streamline your workflow and ensure consistent environments across your team. This guide covers the best practices and tips for effectively using Docker for local development.
1. Docker Compose
Docker Compose is a tool for defining and running multi-container Docker applications. It uses a YAML file to configure your application's services.
Basic Usage
docker-compose up
Multi-stage Builds
Multi-stage builds allow you to build Docker images in multiple stages. This is useful for optimizing the build process and reducing the final image size.
FROM node:18-slim AS builder
WORKDIR /app
COPY package.json package-lock.json ./
RUN npm install
FROM node:18-slim AS final
WORKDIR /app
COPY --from=builder /app /app
RUN npm run build
FROM node:18-slim
WORKDIR /app
COPY --from=final /app /app
RUN npm install
2. Docker Volumes
Docker volumes allow you to persist data and share files between containers.
Basic Usage
docker volume create my-volume
Persistent Data
Use volumes for persistent data:
docker run -v my-volume:/app my-image
3. Docker Networks
Docker networks allow you to connect containers together.
Basic Usage
docker network create my-network
Container Networking
Use networks for container networking:
docker run --network my-network my-image
4. Docker Images
Docker images are the building blocks of Docker containers.
Basic Usage
docker pull node:18-slim
Multi-stage Builds
Use multi-stage builds to optimize image size:
docker build -t my-image .
5. Docker Containers
Docker containers are the runtime instances of Docker images.
Basic Usage
docker run node:18-slim
Multi-stage Builds
Use multi-stage builds to optimize container size:
docker run --rm -v $(pwd):/app -w /app node:18-slim
6. Docker Registries
Docker registries are repositories for Docker images.
Basic Usage
docker pull node:18-slim
Private Registries
Use private registries for secure image storage:
docker pull my-private-registry/node:18-slim
7. Docker Swarm
Docker Swarm is a container orchestration tool that allows you to manage and scale Docker containers across multiple hosts.
Basic Usage
docker swarm init
Scaling Services
Scale services across multiple hosts:
docker service scale my-service=3
8. Docker Compose
Docker Compose is a tool for defining and running multi-container Docker applications. It uses a YAML file to configure your application's services.
Basic Usage
docker-compose up
Multi-stage Builds
Multi-stage builds allow you to build Docker images in multiple stages. This is useful for optimizing the build process and reducing the final image size.
FROM node:18-slim AS builder
WORKDIR /app
COPY package.json package-lock.json ./
RUN npm install
FROM node:18-slim AS final
WORKDIR /app
COPY --from=builder /app /app
RUN npm run build
FROM node:18-slim
WORKDIR /app
COPY --from=final /app /app
RUN npm install
Best Practices
-
Use Custom Properties
<div class="bg-[--custom-bg] text-[--custom-text]"> <!-- Content --> </div>
-
Create Component Classes
@layer components { .btn-primary { @apply px-4 py-2 bg-primary-600 text-white rounded-lg hover:bg-primary-700 transition-colors; } }
-
Maintain Consistent Spacing
<div class="space-y-4 md:space-y-6 lg:space-y-8"> <!-- Vertically spaced content --> </div>
Conclusion
Mastering Docker for local development involves understanding its powerful features and applying them effectively. Remember to:
- Use custom properties
- Create component classes
- Maintain consistent spacing
- Leverage custom properties
Keep exploring the Docker documentation and community resources for more advanced techniques and updates.
Happy coding! 🐳